This guide will take you through the steps needed to integrate OpenAI with your application, get your API key, add funds (if necessary), and understand how the free tier works.
Step 1: Create an OpenAI Account
If you don’t have an OpenAI account yet, follow these steps:
- Go to the OpenAI Platform.
- Click on Sign Up in the top-right corner.
- Follow the instructions to create an account using your email or via Google/Microsoft.
- Once signed up, verify your email to complete the process.
Step 2: Generate Your OpenAI API Key
The API key is essential to authenticate your OpenAI requests. Here’s how to get it:
- Log in to your OpenAI account.
- Go to the API Keys page: https://platform.openai.com/account/api-keys.
- Click on Create new secret key.
- A key will be generated. Copy it and store it securely. You won’t be able to view it again after leaving the page.
- Note: This key is crucial for API access. Do not share it publicly or hardcode it into unsecured code. Instead, use environment variables to manage it securely in your application.
Step 3: Integrating OpenAI With Bevatel
Once you have your API key, you can integrate OpenAI into your application or platform by following these steps:
- Log in to the Bevatel.
- Go to the Integrations module in the sidebar.
- Click on OpenAI and press Connect.
- Paste your OpenAI API key into the provided field.
- Click Create to complete the integration.
Now, your platform should be connected with OpenAI, allowing you to leverage GPT models for generating AI-based responses, completing tasks, or other capabilities provided by OpenAI.
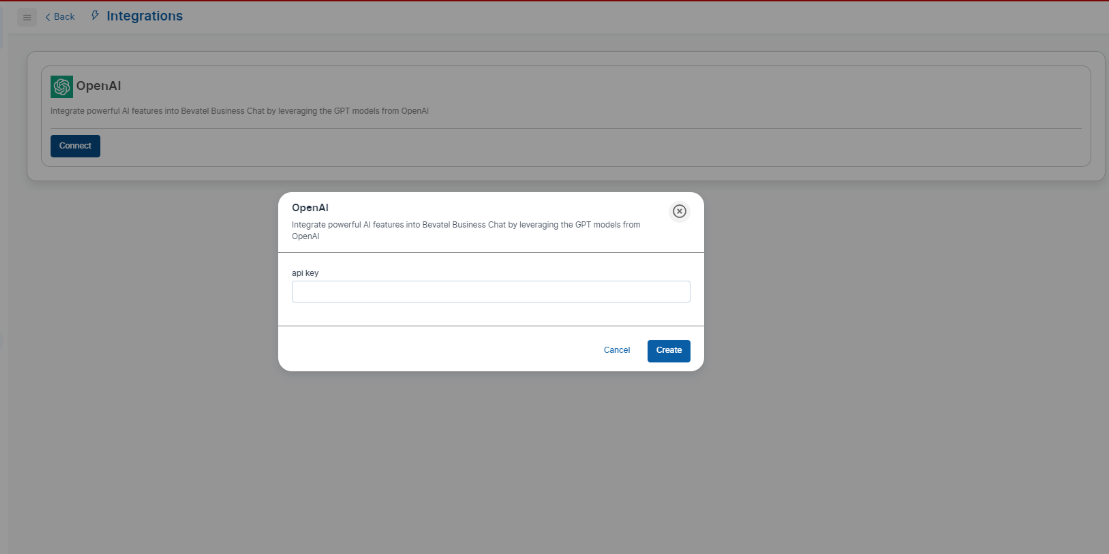
Step 4: Adding Funds to Your Account
Once your free credits run out or if you require more extensive usage, you’ll need to add funds to your OpenAI account. Here’s how:
- Log in to your OpenAI account.
- Navigate to the Billing page: https://platform.openai.com/account/billing.
- Add a payment method, such as a credit card, by selecting Add Payment Method.
- After adding a payment method, you can view your balance and usage.
- OpenAI allows you to set usage limits and spending caps to prevent accidental overuse.
Monitoring Usage and Spending
- You can keep track of your usage on the Usage page.
- Set monthly usage limits to ensure you stay within your desired budget.
You’ll be billed only for the API requests you make that go beyond the free tier limits
Step 5: Understanding the OpenAI Free Tier
OpenAI offers a free tier that allows you to test and use their services without immediate charges. Here’s what you need to know:
1. Free Credits for New Users
- When you sign up, you’ll receive $5 worth of free credits.
- These credits are valid for 3 months from the date of your account creation.
- You can use these credits to explore different OpenAI models and test various APIs.
2. Token-Based Pricing
- OpenAI uses tokens to calculate usage.
- 1 token ≈ 4 characters of English text. A word like "cat" would use one token, while "fantastic" might use two.
- The number of tokens used per request includes both the input prompt and the output generated by the model.
3. Models Available in the Free Tier
- Models like text-davinci-003, text-curie-001, text-babbage-001, and text-ada-001 are available.
- Larger models are more expensive per token but produce more detailed results.
- Smaller models are cheaper and faster but produce less detailed results.
- You can also experiment with models for code generation, speech-to-text (Whisper), and image generation, depending on your needs.
4. Free Tier Usage Limits
- OpenAI offers a limited amount of free usage every month, even after your initial credits expire. You can continue making requests up to a set number of tokens before you need to add payment.
- Exact limits and usage details are updated frequently, so check OpenAI’s pricing page for the most up-to-date information.
.
Sample Code for Using Your OpenAI Key
Once the integration is complete, you can start using OpenAI in your code. Here’s an example in Python:
python
import openai
# Set your OpenAI API key
openai.api_key = "your-secret-api-key"
# Make a request to the OpenAI API
response = openai.Completion.create(
model="text-davinci-003",
prompt="Translate this sentence into French: 'How are you today?'",
max_tokens=60
)
# Print the response
print(response.choices[0].text.strip())
Replace "your-secret-api-key" with your actual API key.
Common Issues and Troubleshooting
Issue 1: API Key Not Working
- Ensure that the API key is correct and hasn't been deleted in your OpenAI account.
- Ensure your code is accessing the key securely (use environment variables).
Issue 2: Hitting Rate Limits
- OpenAI imposes rate limits on the number of requests you can make per minute. You can check your current limits in the Usage section of your OpenAI account.
- If needed, you can request a rate limit increase from OpenAI.
Issue 3: Billing and Usage Problems
- If you encounter issues with payment, check your Billing page for errors with the payment method.
- Make sure your usage limit is appropriately set to avoid overuse charges.
Conclusion
By following the steps in this guide, you’ll be able to:
- Generate and secure your OpenAI API key.
- Integrate OpenAI with your platform.
- Take advantage of OpenAI’s free tier for initial testing and usage.
- Add funds and monitor usage when scaling up your application.